What Is Shell Scripting in Linux and How to Get Started?
Shell scripting in Linux involves writing scripts to automate tasks, manage systems, and streamline workflows. Scripts are text files containing commands executed by a shell interpreter like Bash. Key features include variables, loops, and conditional statements. Real-world applications range from backups to server monitoring. To get started, create a script with a shebang, write commands, make it executable, and run it. Shell scripting is a must-have skill for Linux users, enabling efficiency and automation.
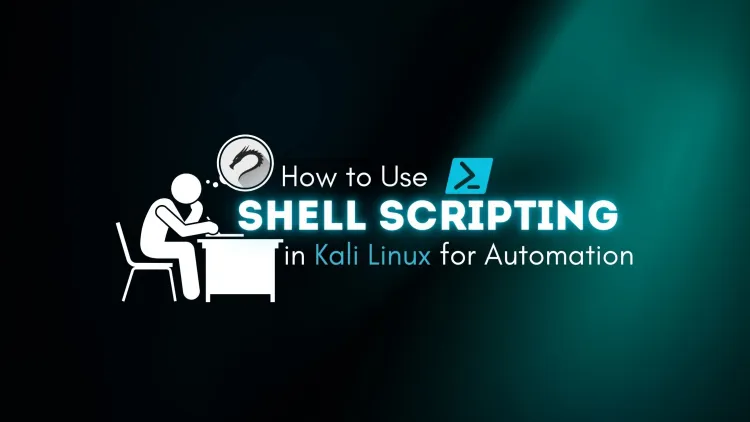
Shell scripting is a powerful tool in Linux that allows users to automate tasks, manage system operations, and streamline workflows. By writing a series of commands in a file and executing them sequentially, shell scripts can save time and reduce repetitive tasks. Whether you are a beginner or an experienced Linux user, shell scripting is an essential skill to master.
What Is Shell Scripting?
At its core, shell scripting involves writing a script—a text file containing a sequence of shell commands—to be executed by a shell interpreter like Bash, Zsh, or Ksh. The shell acts as a bridge between the user and the operating system, interpreting commands and executing them.
- Key Features of Shell Scripting:
- Automates repetitive tasks.
- Simplifies complex commands by bundling them into a single script.
- Enhances system administration and management.
- Supports conditional statements, loops, and functions for advanced logic.
Why Use Shell Scripting?
Shell scripting is widely used in Linux for the following reasons:
- Automation: Streamlines repetitive tasks like backups, monitoring, or updates.
- System Administration: Manages user accounts, file permissions, and server configurations.
- Customization: Adapts the environment to specific requirements by automating custom workflows.
- Efficiency: Reduces manual errors and saves time with batch processing.
Getting Started with Shell Scripting
1. Basics of Shell Scripting
-
Create a Shell Script:
A shell script is simply a text file with executable permissions.- Example: Create a file named
script.sh
:
- Example: Create a file named
-
Add a Shebang Line:
The first line of every shell script is the shebang, which specifies the shell to use. -
Write Your Commands:
Add Linux commands below the shebang. For example: -
Make the Script Executable:
Change the file permissions to make the script executable: -
Run the Script:
Execute the script using:
2. Common Shell Scripting Constructs
Variables
Variables store data for reuse within a script.
Conditional Statements
Execute commands based on conditions.
Loops
Automate repetitive tasks with loops.
- For Loop:
- While Loop:
Functions
Group reusable code into functions.
3. Debugging Shell Scripts
Use debugging options to identify and resolve errors:
- Run the script in debug mode:
- Add debugging flags in the script:
4. Best Practices for Shell Scripting
- Use Comments: Document your script with comments for clarity.
- Error Handling: Use
set -e
to stop the script if any command fails. - Modular Design: Use functions to organize code logically.
- Validate Input: Always validate user input to avoid unexpected behavior.
Real-World Applications of Shell Scripting
-
Automated Backups:
Create daily backups of critical files. -
Server Monitoring:
Check disk usage and send alerts. -
User Management:
Automate the creation of user accounts.
Popular Shell Interpreters
- Bash (Bourne Again Shell): The most widely used shell, known for its simplicity and features.
- Zsh (Z Shell): Enhanced with better completion and customization.
- Ksh (Korn Shell): Offers advanced scripting capabilities.
- Fish (Friendly Interactive Shell): User-friendly and interactive, ideal for beginners.
Conclusion
Shell scripting in Linux is a versatile and powerful skill that enhances productivity and automates complex tasks. Whether you're managing systems, customizing workflows, or developing automated solutions, mastering shell scripting can significantly improve your Linux experience. Start small, experiment with commands, and build scripts that make your day-to-day tasks more efficient.
FAQs
-
What is shell scripting in Linux?
- Shell scripting is the process of writing a series of commands in a file, which can be executed by a shell interpreter like Bash, Zsh, or Ksh to automate tasks and manage systems efficiently.
-
Why should I learn shell scripting?
- Shell scripting helps automate repetitive tasks, manage system operations, reduce manual errors, and save time, making it an essential skill for Linux system administrators and developers.
-
What is a shebang (
#!
) in shell scripting?- A shebang (
#!/bin/bash
) is the first line of a script, specifying the shell interpreter to use for executing the script.
- A shebang (
-
How do I create and run a shell script?
- To create a script:
- Use a text editor like
nano
orvim
to write commands in a file (e.g.,script.sh
). - Add a shebang at the top.
- Save the file, make it executable (
chmod +x script.sh
), and run it (./script.sh
).
- Use a text editor like
- To create a script:
-
What are variables in shell scripting?
- Variables are used to store data for reuse within a script. For example:
-
Can I use loops and conditional statements in shell scripting?
- Yes, loops (
for
,while
) and conditionals (if
,case
) are integral parts of shell scripting for executing tasks based on conditions or in iterations.
- Yes, loops (
-
What are some common applications of shell scripting?
- Common uses include:
- Automated backups
- Server monitoring
- Batch file processing
- User account management
- Common uses include:
-
How can I debug shell scripts?
- Use the
bash -x script.sh
command or includeset -x
within the script to enable debugging and identify errors.
- Use the
-
What is the difference between Bash and other shells like Zsh or Ksh?
- While Bash is the most common shell, Zsh offers better auto-completion and customization, and Ksh provides advanced scripting features. Choose the shell based on your requirements.
-
What are the best practices for shell scripting?
- Use comments for clarity.
- Handle errors with
set -e
. - Validate user inputs.
- Follow modular programming by using functions.
- Regularly test and debug scripts to ensure reliability.