Mastering Bash Scripting | Automate Linux Tasks Like a Pro with Practical Examples and Commands
Bash scripting is a powerful way to automate tasks in Linux, making system administration, development, and security tasks more efficient. This blog provides an in-depth guide to Bash scripting, covering its fundamentals, real-world applications, common commands, loops, functions, and best practices. Readers will learn how to write and execute Bash scripts to streamline repetitive tasks. The blog also includes practical examples, tables, and useful Bash commands to help users become proficient in Linux automation. Whether you are a beginner or an advanced Linux user, mastering Bash scripting can greatly improve productivity.
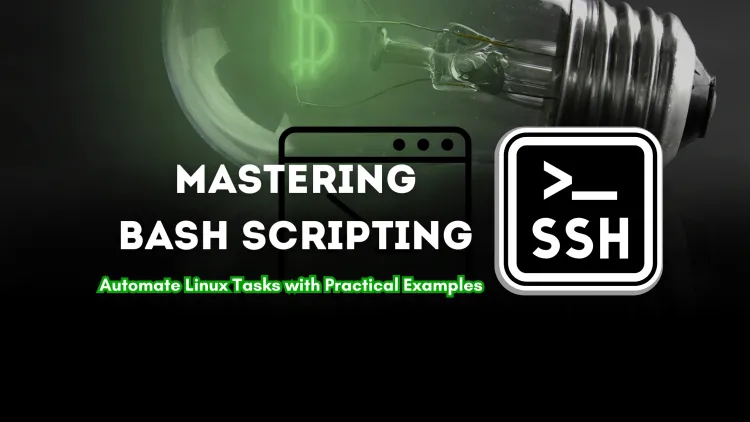
Table of Contents
- Introduction to Bash Scripting
- What is Bash Scripting?
- Why Use Bash Scripting?
- Basic Bash Scripting Commands
- How to Write a Bash Script
- Real-World Example: Automating System Updates
- Best Practices for Bash Scripting
- Conclusion
- Frequently Asked Questions (FAQs)
Introduction to Bash Scripting
Bash scripting is a powerful way to automate tasks in Linux, saving time and reducing errors. Whether you are a system administrator, developer, or ethical hacker, learning Bash scripting helps you handle repetitive tasks efficiently.
In this blog, we will cover:
- What Bash scripting is
- Why it is useful
- Common commands and examples
- How to write and run a Bash script
By the end of this blog, you will be able to create and execute Bash scripts like a pro!
What is Bash Scripting?
Bash (Bourne Again Shell) is the default command-line shell in most Linux distributions. A Bash script is a text file containing a series of commands that are executed in sequence. Instead of typing commands manually, you can automate them using a script.
Example of a Simple Bash Script
#!/bin/bash
echo "Hello, World!"
This script simply prints Hello, World! on the screen.
How to Run a Bash Script
- Save the script as
script.sh
- Give it execute permission:
chmod +x script.sh
- Run the script:
./script.sh
Why Use Bash Scripting?
Bash scripting is used for various tasks, such as:
Use Case | Example |
---|---|
Automating backups | Create a script to back up files daily |
User management | Add or remove users automatically |
Log file analysis | Extract data from logs efficiently |
Software installation | Install packages with a single command |
Network monitoring | Check server status and connectivity |
Basic Bash Scripting Commands
Here are some common Bash commands used in scripting:
Command | Description | Example |
---|---|---|
echo |
Prints text to the screen | echo "Hello, Linux" |
ls |
Lists files in a directory | ls /home/user |
cd |
Changes the current directory | cd /var/logs |
mkdir |
Creates a new directory | mkdir new_folder |
rm |
Deletes a file or directory | rm file.txt |
cp |
Copies a file or directory | cp file.txt backup/ |
mv |
Moves or renames a file | mv old.txt new.txt |
cat |
Displays the content of a file | cat notes.txt |
grep |
Searches for a pattern in a file | grep "error" log.txt |
chmod |
Changes file permissions | chmod +x script.sh |
How to Write a Bash Script
1. Using Variables
Variables store values that can be used later in the script.
#!/bin/bash
name="John"
echo "Hello, $name!"
Output:
Hello, John!
2. Using Conditional Statements
Bash supports if-else conditions to make decisions.
#!/bin/bash
read -p "Enter your age: " age
if [ $age -ge 18 ]; then
echo "You are eligible to vote."
else
echo "You are not eligible to vote."
fi
3. Using Loops
Loops are used to repeat tasks multiple times.
For Loop Example
#!/bin/bash
for i in {1..5}
do
echo "Number: $i"
done
Output:
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
While Loop Example
#!/bin/bash
count=1
while [ $count -le 3 ]
do
echo "Count: $count"
((count++))
done
4. Using Functions
Functions allow you to reuse code within a script.
#!/bin/bash
greet() {
echo "Welcome, $1!"
}
greet "Alice"
greet "Bob"
Output:
Welcome, Alice!
Welcome, Bob!
Real-World Example: Automating System Updates
This script automatically updates the system and cleans up unnecessary files.
#!/bin/bash
echo "Updating system..."
sudo apt update && sudo apt upgrade -y
echo "Cleaning up..."
sudo apt autoremove -y
echo "Update complete!"
This script is useful for system administrators to keep servers up to date.
Best Practices for Bash Scripting
- Always start with
#!/bin/bash
to specify the script interpreter. - Use comments (
#
) to explain each part of the script. - Use descriptive variable names for readability.
- Handle errors using
if
conditions. - Test scripts in a safe environment before deploying them.
Conclusion
Bash scripting is an essential skill for Linux users. It helps in automating tasks, managing systems efficiently, and reducing manual effort. Whether you are a beginner or an experienced user, learning Bash scripting can significantly improve your workflow.
Start practicing today and become a Bash scripting pro!
Frequently Asked Questions (FAQs)
What is Bash scripting?
Bash scripting is the process of writing a sequence of Linux commands in a file to automate repetitive tasks.
Why should I learn Bash scripting?
Bash scripting helps automate system tasks, improves efficiency, and reduces human error in Linux administration.
How do I create a Bash script?
You can create a Bash script using a text editor, save it with a .sh
extension, and make it executable using chmod +x script.sh
.
How do I run a Bash script?
You can run a Bash script by using ./script.sh
in the terminal after making it executable.
What is the purpose of #!/bin/bash
at the beginning of a script?
It specifies the interpreter (Bash shell) that should execute the script.
What are variables in Bash scripting?
Variables store values that can be reused in a script, such as name="Alice"
and echo $name
.
How do I take user input in a Bash script?
You can use the read
command, for example:
What is an if-else statement in Bash?
An if-else statement is used for decision-making based on conditions.
What are loops in Bash scripting?
Loops repeat a set of commands multiple times, such as for
, while
, and until
loops.
How does a for loop work in Bash?
A for loop iterates over a range of values:
What is a while loop in Bash scripting?
A while loop runs until a condition becomes false:
How can I write functions in Bash?
Functions help reuse code inside a script:
What is the difference between echo
and printf
in Bash?
echo
prints text with a newline, while printf
allows formatted output.
How can I pass arguments to a Bash script?
You can pass arguments using $1
, $2
, etc. Example:
What is the use of chmod +x script.sh
?
It makes the script executable so that it can be run from the terminal.
How can I schedule a Bash script to run automatically?
You can use cron jobs (crontab -e
) to schedule scripts at specific times.
What is the difference between >
and >>
in Bash?
>
overwrites a file, while >>
appends to a file.
How can I debug a Bash script?
Run the script with bash -x script.sh
to see step-by-step execution.
What is a case statement in Bash scripting?
A case
statement is an alternative to multiple if-else conditions.
Can I use Bash scripting for automation in cybersecurity?
Yes, ethical hackers use Bash scripting to automate tasks like network scanning and log analysis.
How do I handle errors in a Bash script?
Use set -e
to stop execution on errors or ||
to handle failures gracefully.
What is the purpose of export
in Bash?
export
makes a variable available in child processes.
How can I check if a file exists in Bash?
Use the -f
flag:
What is the use of awk
and sed
in Bash?
awk
and sed
are used for text processing in scripts.
How do I comment in Bash scripts?
Use #
for single-line comments.
How can I create a log file in Bash?
Redirect script output to a log file using:
How can I find and replace text in a file using Bash?
Use sed
:
How do I loop through a list of files in a directory?
Use a for
loop:
How do I kill a running process using Bash?
Use kill
followed by the process ID (PID):
What are the best practices for writing Bash scripts?
- Always use comments to explain the script
- Test your script before deploying it
- Handle errors properly
- Use meaningful variable names
- Avoid hardcoded values, use variables instead